Part can use rich controllers provided by Adichatz as GMapController.
Before using GMapController, get GMap API key and store it in './resources/xml/AdichatzRcpConfig.xml' file as shown below.
<?xml version="1.0" encoding="UTF-8" standalone="yes"?> <adichatzRcpConfigTree xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="http://www.adichatz.org/xsd/v0.9.0/engine/adichatzRcpConfigTree.xsd"> <rcpConfiguration> ... <param id="adichatzGMapAPIKey" value="YOUR_GMAP_API_KEY"/> ... </rcpConfiguration> </adichatzRcpConfigTree>
For example, open file $projectDirectory/resources/xml/model/address/AddressDIGENERATED.xml.
Following XML elements:
<?xml version="1.0" encoding="UTF-8" standalone="yes"?> <?xml version="1.0" encoding="UTF-8" standalone="yes"?> <includeTree xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" coreClassName="org.adichatz.engine.core.ASectionCore" entityURI="adi://myproject/model.actor/ActorMM" xsi:noNamespaceSchemaLocation="http://www.adichatz.org/xsd/v0.9.1/generator/includeTree.xsd"> <section text="#MSG(actor, detailContainerText)" id="detailContainer"> <layout layoutConstraints="wrap 4" columnConstraints="[fill, align right]10[fill,grow]25[align right]10[fill,grow]"/> <include adiResourceURI="getToolBarURI((String) #PARAM(TOOL_BAR_TYPE))" id="detailToolbarMenu"> <params> <param value="#CONTROLLER(detailContainer)" id="CONTROLLER"/> <param optional="true" value="#PARAM(TOOL_BAR_TYPE)" id="TOOL_BAR_TYPE"/> </params> </include> <formattedText editPattern="#####" format="Integer" property="actorId" enabled="false" id="actorId"/> <text textLimit="45" property="firstName" mandatory="true" id="firstName"/> <text textLimit="45" property="lastName" mandatory="true" id="lastName"/> <dateText property="lastUpdate" enabled="false" style="SWT.BORDER | SWT.TIME" id="lastUpdate"/> </section> </includeTree>
renders the following layout:
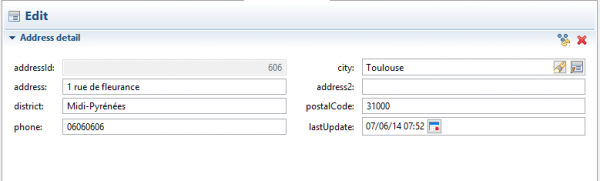
Change XML elements:
- Copy AddressDIGENERATED.axml file to AddressDI.axml which will become the reference for generated code.
- Replace above XML lines with the following ones:
<?xml version="1.0" encoding="UTF-8" standalone="yes"?> <includeTree xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" coreClassName="org.adichatz.engine.core.ASectionCore" entityURI="adi://myproject/model.address/AddressMM" generationType="DETAIL" xsi:noNamespaceSchemaLocation="http://www.adichatz.org/xsd/v0.8.4/generator/includeTree.xsd"> <section text="#MSG(actor, detailContainerText)" id="detailContainer"> <layout layoutConstraints="wrap 2" columnConstraints="[fill]25[fill,grow]" rowConstraints="[fill, grow]"/> <include adiResourceURI="getToolBarURI((String) #PARAM(TOOL_BAR_TYPE))" id="detailToolbarMenu"> <params> <param value="#CONTROLLER(detailContainer)" id="CONTROLLER"/> <param optional="true" value="#PARAM(TOOL_BAR_TYPE)" id="TOOL_BAR_TYPE"/> </params> </include> <listeners> <listener listenerTypes="AFTER_SYNCHRONIZE | AFTER_PROPERTY_CHANGE | POST_REFRESH"> <code>displayGMap();</code> </listener> </listeners> <additionalCode>import org.adichatz.engine.widgets.GMap; import java.lang.Runnable; private boolean doit = true; private void displayGMap() { if (doit) { doit = false; GMap gmapControl = #CONTROL(map); Address address = #BEAN(); if (null != address) { StringBuffer addresSB = new StringBuffer(); if (null != address.getAddress()) addresSB.append(address.getAddress()).append(" "); if (null != address.getAddress2()) addresSB.append(address.getAddress2()).append(" "); if (null != address.getPostalCode()) addresSB.append(address.getPostalCode()).append(" "); if (null != address.getCity()) { addresSB.append(address.getCity().getCity()).append(" "); addresSB.append(#BEAN().city.country.country); } gmapControl.setValue(addresSB.toString().trim()); } else gmapControl.setValue(null); doit = true; } }</additionalCode> <composite> <layout layoutConstraints="wrap 2" columnConstraints="[fill, align right]10[grow,fill]" rowConstraints="20[]20[][]"/> <formattedText editPattern="######" format="Short" property="addressId" enabled="false" id="addressId"/> <refText property="city" mandatory="true" style="SWT.BORDER | AdiSWT.FIND_BUTTON | AdiSWT.EDITOR_BUTTON" id="city"> <convertModelToTarget>return null==value ? "" : #FV().city;</convertModelToTarget> </refText> <text textLimit="50" property="address" mandatory="true" id="address"/> <text textLimit="50" property="address2" id="address2"/> <text textLimit="20" property="district" mandatory="true" id="district"/> <text textLimit="10" property="postalCode" id="postalCode"/> <text textLimit="20" property="phone" mandatory="true" id="phone"/> <dateText property="lastUpdate" enabled="false" style="SWT.BORDER | SWT.TIME" id="lastUpdate"/> </composite> <gMap mapDataType="ADDRESS" toolBarStyle="AdiSWT.EXPANDABLE" zoom="12" id="map" delayMillisLoading="500"/> </section> </includeTree>renders the new layout:
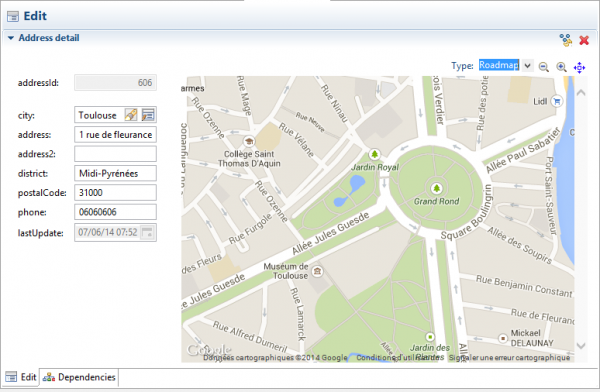
Remarks:
Lines 12-14: Method displayGMap is called at three moments of the life cycle of the controller:
- AFTER_SYNCHRONIZE: After received entity is injected inside UI controllers.
- AFTER_PROPERTY_CHANGE: After UI send a change to a property of the entity.
- POST_REFRESH: After changes on entity are cancelled by asking to refresh property values from Database.
Lines 16-41: Build an address string from property values and set the string to the GMap control.
Line 55: Set a gmap controller.